20 Copy Array Of Objects Javascript
In an earlier article, we looked at different ways to make a copy of an array in JavaScript. However, all these methods only create a shallow copy of the original array. This means that if the original array contains objects, only their references will be passed to the new array. 14/5/2020 · Arrays of objects don't stay the same all the time. We almost always need to manipulate them. So let's take a look at how we can add objects to an already existing array. Add a new object at the start - Array.unshift. To add an object at the first position, use Array.unshift.
Find A Value In An Array Of Objects In Javascript Stack
I had to use this thing to create a copy of the array with objects, but objects would be stripped of like 12 unnecessary keys before they are parsed by my other functions. Using it for filtering the array, that is considerably large. This does the job well so far.
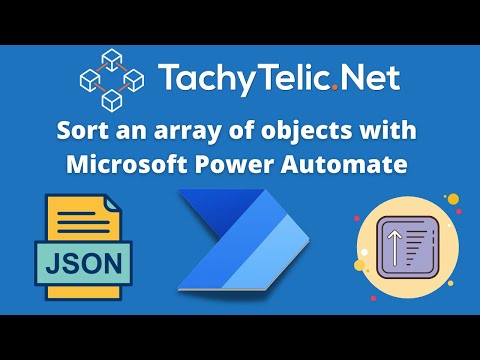
Copy array of objects javascript. Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: Clone an Array in JavaScript You don't need to iterate over the original array and push each item into another array. Cloning arrays in JavaScript is as straightforward as slice or concat. Calling.slice on the original array clones the array: Before starting to learn the method of copying, we have to clarify the two-term shallow copy and the deep copy. Shallow copy only copies the first level element. That's mean it doesn't work for the multi-dimension array. Those are pass as a reference. But deep copy will copy the first level as well as a nested element to the new array.
Extract Data from Arrays and Objects in JavaScript. In this blog, we are going to explore how to extract data from arrays and objects in javaScript using destructuring. JavaScript destructuring assignment is another feature deployed as a part of the ECMAScript release in 2015. Arrays are mutable typ e in Javascript! That means the value of the array can be changed after it has been declared. In this case array2 is copying the reference of array1. This is not a shallow ... JavaScript offers many ways to create shallow and deep clones of objects. You can use the spread operator (...) and Object.assign () method to quickly create a shallow object duplicate. For the deep cloning of objects, you can either write your own custom function or use a 3rd-party library like Lodash.
In javascript, When creating copies of arrays or objects one can make a deep copy or a shallow copy. so we will see what's deep copy and shallow copy, and how to handle this problem. Deep copy or... Note: This also assigns objects/arrays by reference instead of by value. 10. Array.from (Shallow copy) This can turn any iterable object into an array. Giving an array returns a shallow copy. numbers = [1, 2, 3]; numbersCopy = Array.from(numbers); // [1, 2, 3] Note: This also assigns objects/arrays by reference instead of by value. Conclusion ... This method is another popular way to copy an array in Javascript. const originalArray = [1,2,3,4,5] const clone = [].concat(originalArray) Concat is a very useful method to merge two iterable. In this way, we take an empty array and concatenate the original array into it. It creates a fresh copy of the array. Mutable vs Immutable Objects Javascript
In case a property inside the original object is an object, it can be shared between the copy and the original one. Notice that by nature, JavaScript objects are changeable and are stored as a reference. So, when assigning the object to another variable, you assign the memory address of the object to the variable. If you looking for a better understanding of objects, arrays and their references and creating a copy of them then you are at the right place. Javascript Objects and Arrays are mutable, which means their state can be modified after it is being created. The slice () method returns a shallow copy of a portion of an array into a new array object selected from start to end (end not included) where start and end represent the index of items in that array. The original array will not be modified.
To generalize this into a function, do the following: function pushArray(arr, arr2) { arr.push.apply (arr, arr2); console .log (arr); } pushArray ( [ 1, 2 ], [ 3, 4 ]); How to Copy Array Items into Another Array. How to Copy Array Items into Another Array. Photo by Landon Martin on Unsplash What is a deep copy? F or objects and arrays containing other objects or arrays, copying these objects requires a deep copy. Otherwise, changes made to the nested references will change the data nested in the original object or array. This is compared to a shallow copy, which works fine for an object or array containing only primitive values, but will fail ... Shallow copyingonly copies the top-level entries of objects and Arrays. The entry values are still the same in original and copy. Deep copyingalso copies the entries of the values of the entries, etc. That is, it traverses the complete tree whose root is the value to be copied and makes copies of all nodes.
JavaScript map with an array of objects. JavaScript map method is used to call a function on each element of an array to create a different array based on the outputs of the function. It creates a new array without modifying the elements of the original array. 4/5/2017 · In JavaScript, array and object copy change the original values, so a deep copy is the solution for this. A deep copy means actually creating a new array and copying over the values, since whatever happens to it will never affect the origin one. JSON.parse and JSON.stringify is the best and simple way to deep copy. # How to Deep Clone an Array. There are 2 types of array cloning: shallow & deep. Shallow copies only cover the 1st level of the array and the rest are referenced. If you want a true copy of nested arrays, you'll need a deep clone. For deep clones, go with the JSON way OR better yet use Lodash 👍
Methods of JavaScript Copy Array. In this article, we will study all the three methods of copying an array and also see the working of the equal to and other methods in a comparative manner that will get you a clear perspective of using these methods to copy an array and look at some of the examples and syntax. 1. Shallow copy. To shallow copy, an object means to simply create a new object with the exact same set of properties. We call the copy shallow because the properties in the target object can still hold references to those in the source object.. Before we get going with the implementation, however, let's first write some tests, so that later we can check if everything is working as expected. That means we need to not just copy the objects to a new array (or a target array), but also create copies of the objects. If the destination array doesn't exist yet......use map to create a new array, and copy the objects as you go: const newArray = sourceArray.map (obj => /*...create and return copy …
To deep-clone an array of objects in JavaScript, we can first pass the array into the stringify () method on the global JSON object and then use the parse () method on the global JSON object to convert the array string into a valid copy of the same array. Objects are assigned and copied by reference. In other words, a variable stores not the "object value", but a "reference" (address in memory) for the value. So copying such a variable or passing it as a function argument copies that reference, not the object itself. #Lodash DeepClone vs JSON. Here's a comment from the community. Yes, it was for my previous post, How to Deep Clone an Array.But the idea still applies to objects. Alfredo Salzillo: I'd like you to note that there are some differences between deepClone and JSON.stringify/parse.. JSON.stringify/parse only work with Number and String and Object literal without function or Symbol properties.
Shallow copy an array Arrays in javascript are just objects with some additional properties and methods which make them act like an array. So when we directly assign array to another variable it is shallowly copied. This means it is just referencing to the array not copying all the elements. The spread operator in ES6 is used to clone an array, whereas slice () method in JavaScript is an older way that provide 0 as the first argument. These methods create a new, independent array and copy all the elements of oldArray to the new one i.e. both these methods do a shallow copy of the original array. The Rest/Spread Properties for ECMAScript proposal (ES2018) added spread properties to object literals. It copies own enumerable properties from a provided object onto a new object. Shallow-cloning (excluding prototype) or merging of objects is now possible using a shorter syntax than Object.assign ().
If you need a deep copy of an Array of Objects with circular references I believe you're going to have to code your own method to handle your specialized data structure, such that it is a multi-pass clone: On round one, make a clone of all objects that don't reference other objects in the array. Keep a track of each object's origins.
Javascript Array Of Objects Check Duplicates Code Example
How To Copy An Array In Javascript
How To Deep Copy Objects And Arrays In Javascript By Dr
How To Deep Clone Or Copy An Array Of Objects Using The Json
Remove Object From An Array Of Objects In Javascript
Javascript Array A Complete Guide For Beginners Dataflair
How To Clone An Array In Javascript
Javascript Remove Duplicate Objects From Array Es6
9 Ways To Remove Elements From A Javascript Array
Javascript Copy Array Cloning An Array Of Objects In
How To Find Unique Values By Property In An Array Of Objects
3 Ways To Clone Objects In Javascript Samanthaming Com
How To Use The Spread Operator In Javascript
How To Sort An Array Of Objects With Power Automate
How To Deep Clone An Array In Javascript Dev Community
Different Ways To Duplicate Objects In Javascript By
How To Change The Value Of An Object In An Array Javascript
All You Need To Know About Javascript Arrays
Hacks For Creating Javascript Arrays
0 Response to "20 Copy Array Of Objects Javascript"
Post a Comment